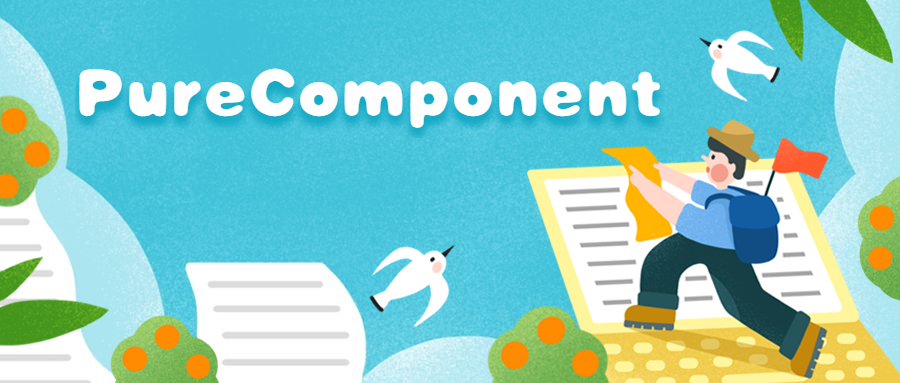
PureComponent 会对 props
和 state
进行浅层比较,并减少了跳过必要更新的可能性。
React.PureComponent
与 React.Component
很相似。
两者的区别在于 React.Component
并未实现 shouldComponentUpdate()
,而 React.PureComponent
中以浅层对比 prop
和 state
的方式来实现了该函数。
如果赋予 React 组件相同的 props
和 state
,render()
函数会渲染相同的内容,那么在某些情况下使用 React.PureComponent
可提高性能。
1 | import React from 'react'; |
上面演示的代码中 Counter
组件中使用了 Title
组件,Counter
组件每次修改状态都会导致 Title
组件重新渲染。可以看到 console
语句多次打印出 Title render
。如果不想让 Title
组件多次渲染,就需要让 Title
组件继承 PureComponent
组件。
开始使用
- 类组件使用
PureComponent
1 | import React from 'react'; |
- 函数组件使用
memo
1 | class Counter extends React.Component { |
memo
的原理
1 | function memo (Func) { |
PureComponent
的原理
1 | import React, { Component } from 'react'; |